Documentation
A decentralized marketplace connecting developers and users through MCP tools, enabling monetization via Recall tokens and creating a seamless ecosystem for specialized AI capabilities.
Overview
Storm is a decentralized marketplace for AI tools where developers can publish and monetize their Model Control Protocol (MCP) tools directly. Consumers access these tools through token-based payments, creating an ecosystem that rewards developers while providing users with diverse AI capabilities.
The platform uses AES encryption for secure tool storage and transmission, and integrates with Recall tokens to enable micropayments between users and developers.
Key Features
Developer Monetization
Publish your MCP tools and earn tokens when consumers use them
Consumer Access
Use a variety of specialized AI tools through a single unified interface
Token Economy
Powered by Recall tokens and wallet integration with account abstraction
Transparent Source Code
Option to showcase tool source code in the frontend
Simple Integration
Easy to install and use with platforms like Claude Desktop
AES Encryption
Industry-standard security for tool parameters and functions
Tool Buckets
Organized storage system for efficient tool management
Decentralized Architecture
Built on Recall Network for censorship-resistant tool distribution
Technical Architecture
Tool Management Flow
- Create Bucket: Initialize storage for new tools
- Add Tool: Encrypt tool parameters and functions with AES before storage
- Retrieve Tool: Securely retrieve and decrypt tools when needed
- MCP Server Integration: Seamlessly connect with MCP servers for tool execution
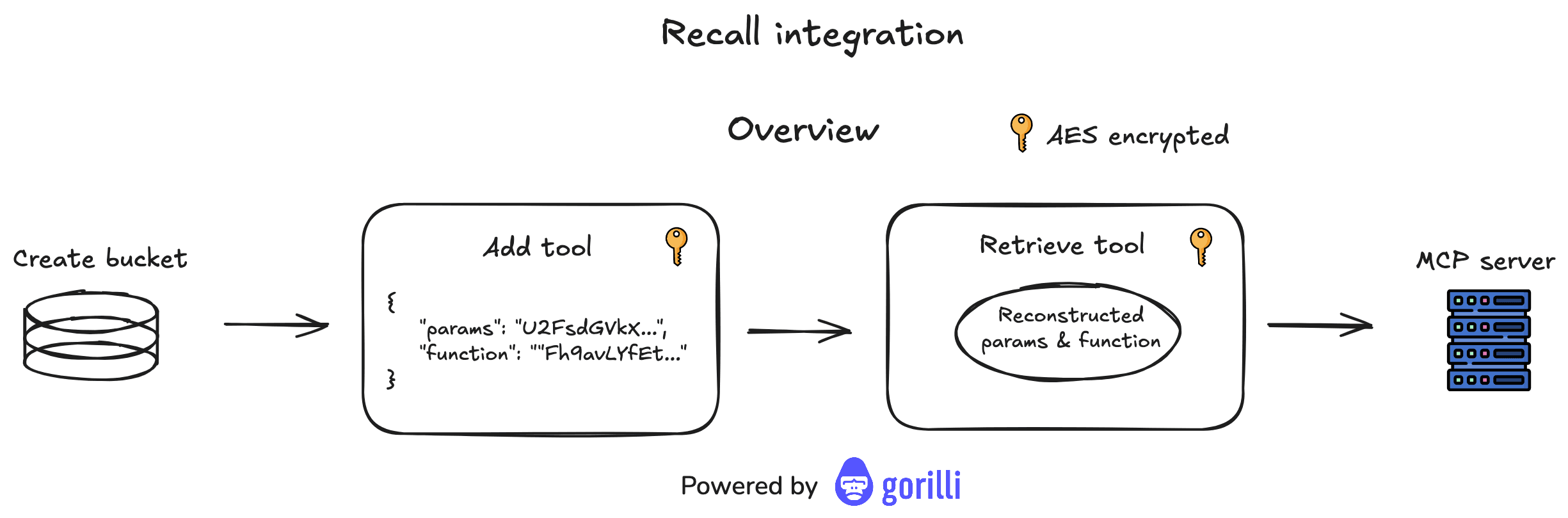
Tool Bucket Structure
- Tool name
- Parameter schemas with validation rules
- Function implementations
- Secure access controls
- Version history
- Usage statistics
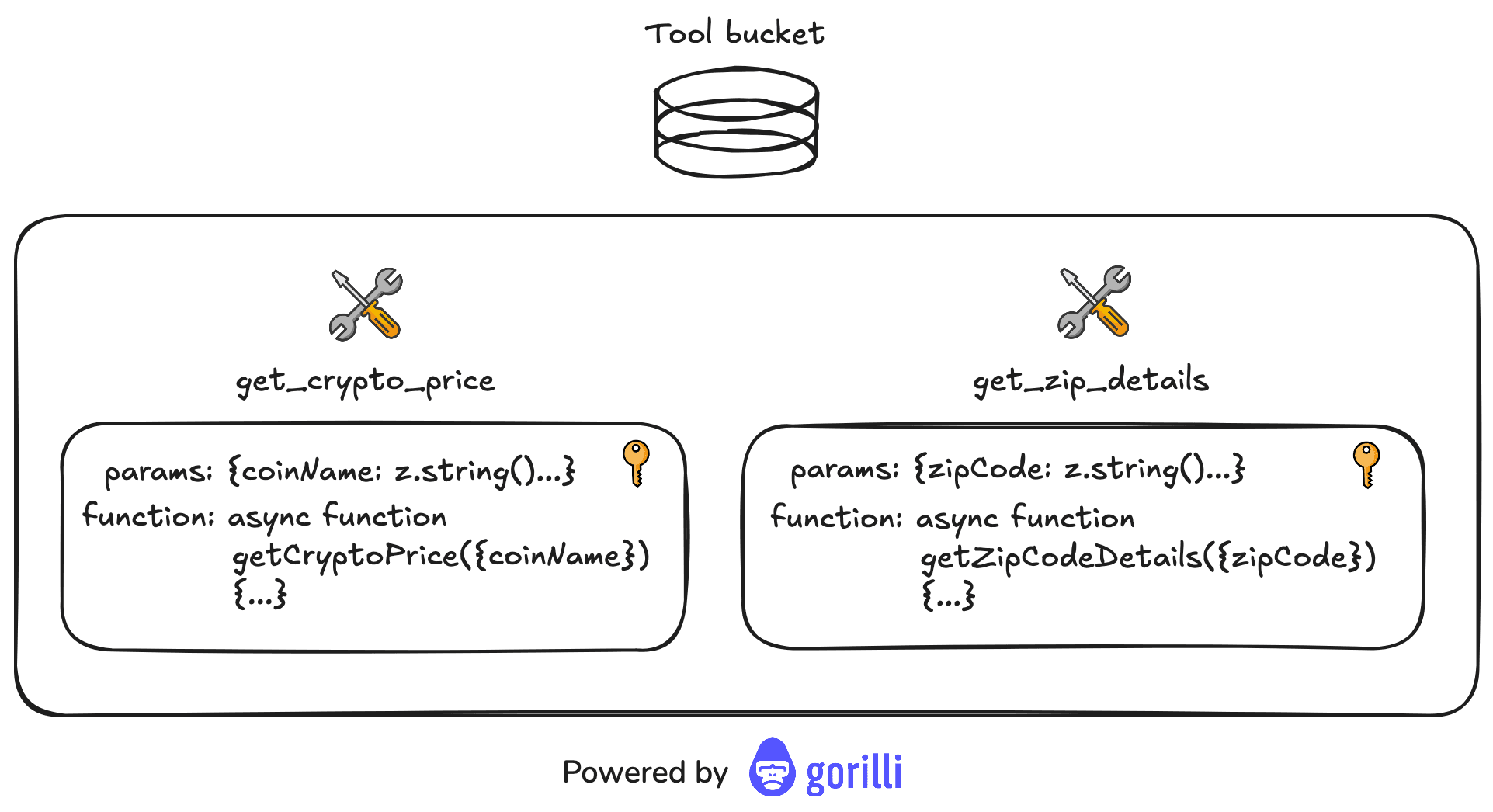
Detailed Tool Processing Workflow
- Parameters and function bodies are separated
- Each component is serialized to JSON/string format
- AES encryption is applied to both components
- The encrypted components are combined into a single object
- The tool is stored in the appropriate bucket
.8dee5775.png&w=3840&q=75)
Tool Retrieval and Execution
- The encrypted tool is retrieved from the bucket
- Decryption is performed using the appropriate keys
- Parameters and functions are reconstructed
- Zod schema validation ensures security and correctness
- The tool is made available to the MCP server for execution
.3647aeac.png&w=3840&q=75)
How It Works
For Developers
- 1Create Your Tool: Develop your MCP-compatible tool using the Storm SDK
- 2Configure Parameters: Define the input parameters and validation rules
- 3Implement Function: Write the core functionality that your tool provides
- 4Publish to Marketplace: Submit your tool with pricing information
- 5Monitor Usage: Track usage statistics and token earnings
For Consumers
- 1Install Storm: Set up the Storm MCP server on your system
- 2Fund Your Wallet: Ensure your wallet has sufficient Recall tokens
- 3Browse Tools: Explore available tools in the marketplace
- 4Use Tools via AI: Make queries to your AI assistant that leverage Storm tools
- 5Automatic Payments: Micropayments are processed automatically to tool developers
Current Tool Types
Security Features
AES Encryption
All tool code and parameters are encrypted at rest and in transit
Secure Key Management
Advanced key rotation and storage
Access Controls
Granular permissions for tool access
Audit Logging
Comprehensive logging of all tool usage
Parameter Validation
Strict schema enforcement using Zod
Smart Contract Security
Audited contracts for token transactions
API Example
import { StormSDK } from "storm-sdk"; const storm = new StormSDK({ apiKey: "your-api-key" }); const myTool = { name: "get_crypto_price", params: { coinName: z.string().min(1).max(50), }, function: async ({ coinName }) => { const price = await fetchCryptoPrice(coinName); return { price, currency: "USD" }; }, }; await storm.publishTool(myTool);